Iterative algorithm coded in Perl
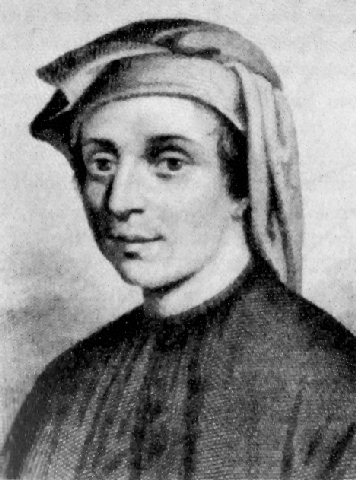
####################################
for ($i=1; $i<=$n; $i++) # by iteration
{
if ($i == 1) # defining the first element
{
$fib_pre_previous = 0;
print "$fib_pre_previous\n";
}
elsif ($i == 2) # defining the second element
{
$fib_previous = 1;
print "$fib_previous\n";
}
else # now each new element is the sum of the previous two
{
$fib_sum = $fib_previous + $fib_pre_previous;
$fib_pre_previous = $fib_previous;
$fib_previous = $fib_sum;
print "$fib_sum\n";
}
}
####################################
Altogether, the iterative algorithm will generate the sequence of 1477 Fibonacci numbers starting from 0 with the largest one being

Very importantly, an iterative algorithm for the Fibonacci sequence generation seems to be the fastest, most accurate, and effective as compared to recursive or arithmetic methods.
In my benchmark test of generating 1475 Fibonacci numbers as many as 1 mln times all over again, the iterative algorithm proved to be 1.5 times faster than the arithmetic one
as evaluated by the wall-clock time consumed by each process.
Now, why don't you go up and challenge the iterative algorithm yourself!